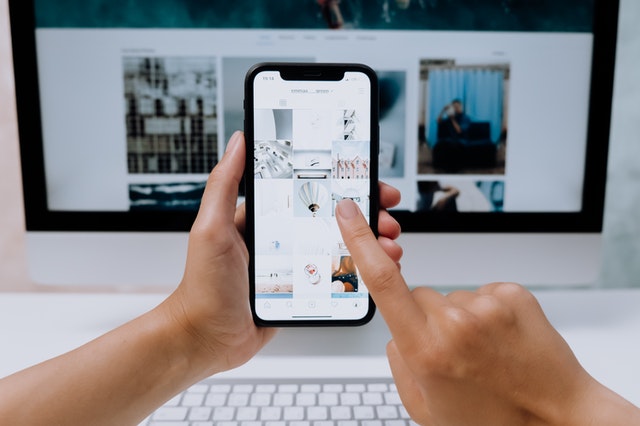
Developing native apps for Android and iOS app with react native using a single code-base is quite simple but publishing can be complicated, particularly with an originally Android-only application.
This article first gives a description of how to get the Android app to work well on iOS and after that how to add a splash screen and app icon.
-
Build up authentication for your react native iOS app:
First, get a free Okta developer account for that install the Okta CLI and run okta register to sign up for a new account. Run okta login if already having an account. Now, run okta apps create. Then select the default app name (or change if you prefer so), choose Native, and press Enter.
Apply com.okta.dev-133337:/callback for the Redirect URI and the Logout Redirect URI. Here, dev-133337.okta.com is an Okta domain name and is reversed to give a unique scheme to open your app on a device.
Okta CLI will generate an OIDC Native App in your Okta Org that adds the specified redirect URIs and permits access to the ‘Everyone’ group. Once it’s finished, the output would be:
Okta application configuration:
Issuer: https://dev-133337.okta.com/oauth2/default
Client ID: 0oab8eb55Kb9jdMIr5d6
Now, find the point at which the config variable is defined in your App.js, generally near the top and change the applicable values to that of your Okta app:
const config = {
issuer: ‘https://{yourOktaDomain}/oauth2/default’,
clientId: ‘{clientId}’,
redirectUrl: ‘{redirectUrl}’,
additionalParameters: {},
scopes: [‘openid’, ‘profile’, ’email’, ‘offline_access’]
};
-
Run the React Native App on iOS simulator:
At first run react-native run-ios from a Mac computer after that, an iOS simulator should appear, and then the project will compile in the console.
Here, you will notice an error ‘AppAuth/AppAuth.h’ file not found, and hence you will have to link the AppAuth library to iOS such as Cocoapods. Now, insert the following into ios/Podfile:
platform :ios, ‘11.0’
target ‘prime_components’ do
pod ‘AppAuth’, ‘>= 0.94’
end
After installing Cocoapods, change into ios/ and run pod install. After this is done, close Xcode and open ios/prime_components.xcworkspace in Xcode. The pods now appear as a separate project. Pick a device and the project will build and run just well after clicking the play button. Remember to change the bundle identifier if the one used here is already taken.
The factorization should work at this point, however, clicking on the Login will lead to crashing as your AppDelegate class should match to RNAppAuthAuthorizationFlowManager. So, now open AppDelegate.h and change it to the following:
#import
#import “RNAppAuthAuthorizationFlowManager.h”
@interface AppDelegate : UIResponder
@property (nonatomic, weak) id
@property (nonatomic, strong) UIWindow *window;
@end
The login button will now take you through the authorization process.
-
Fine-tune styling in your React Native iOS app:
If there is a bit large font in the app or the banner is not very clear then try the following:
In components/Button.js adjust the font size to 25
In components/Header.js adjust the font size to 65
In components/Input.js adjust the flex to 1.5 and the font size to 60
The iOS status bar may create a transparency issue in the header. To overcome this, import StatusBar from react-native in App.js and add
return (
And now the app looks correct.
-
Place the icon and display name and run on a device:
You can use an online service like MacAppIcon for all the required sizes, after having an icon. Then open the prime_components project in Xcode and click on Images.xcassets. With this, you can see all the icons to fill it, simply drag them from Finder.
In the Identity section of the project settings, you can also change the display name of your project to finalize the app name on your device.
Get a set up the signing team and also make sure that the ‘Build Active Architectures Only’ is set to ‘Yes’ for debug as well as release, for both projects. It helps in fixing a lot of integration issues with the AppAuth library.
It makes you to deploy to a device with a proper icon and name for your app.
-
Build a splash screen for your React Native iOS apps:
While loading, iOS apps have splash screens. React Native generates a basic LaunchScreen.dib image which is only a white screen with the app’s name. You can change it by using the React Native Toolbox. Here, you should create a square image of at least 2208×2208 pixels and keep plenty of margin around your symbol.
Now, install the toolbox and ImageMagick:
npm install -g [email protected] [email protected]
brew install imagemagick
Then, put the image inside the project, secure the workspace inside of XCode and run this command; yo rn-toolbox:assets –splash image.png –ios
Specify the correct project name only! This generates images and updates your project. Now, uninstall the app from the simulator/device and re-deploy from Xcode, and a new splash when loading the app will be there.
Lastly, don’t forget to submit your React Native App to the iOS store for which you need to consider elements such as review guidelines, app store connect, build an archive, screenshots, privacy policy, and submission.
Hope the article helps you for building iOS app with React Native. Alternatively, you can also hire a React Native developer to get everything smoothly.